In this part of the lab, you
will work with two categories of
employee, a salaried worker and an hourly worker. The HR.java
is your test driver for this application.
4 Looking for patterns:
This part of the lab will help
you understand why we might
want to use inheritance.
Look at the SalariedWorker
and
HourlyWorker
classes:
- What
attributes are the same
between the Salaried and
Hourly workers? Are there any that are different?
- What
methods are the same in name
between the Salaried and
Hourly workers?
- Which
methods do the same thing?
Which methods do
different things?
- Open both files for editing.
The Payroll department has
decided to add a new field, homeDepartment (a String) to the worker
classes and to print out that new field as part of the toString which
shows the payroll amount. Add this field as an instance variable.
- Alter the constructors to
accept this value as a parameter
and to store it in the new field.
- Alter the toString methods
to print this value directly
following the SSN of the employee.
- Compile and test your
solution, altering the HR program to
pass a department name into the constructor.
- How
many individual statements did
you need to add to SalariedWorker and HourlyWorker collectively?
- Alter the hourly worker pay
method to calculate the pay as follows: (pays time and 1/2 for hours
over 80).:
if(hours > 80)
payAmt = (hours * rate) + ((hours - 80) * .5 * rate);
else
payAmt = hours * rate;
- Test this by passing in a
larger number
of hours to one of the two hourly worker's pay method. Correct any defects.
- Did this change require
any changes to your SalariedWorker class?
5 A better solution:
This part of the lab will help you to understand what inheritance in
java is and how you implement inheritance.
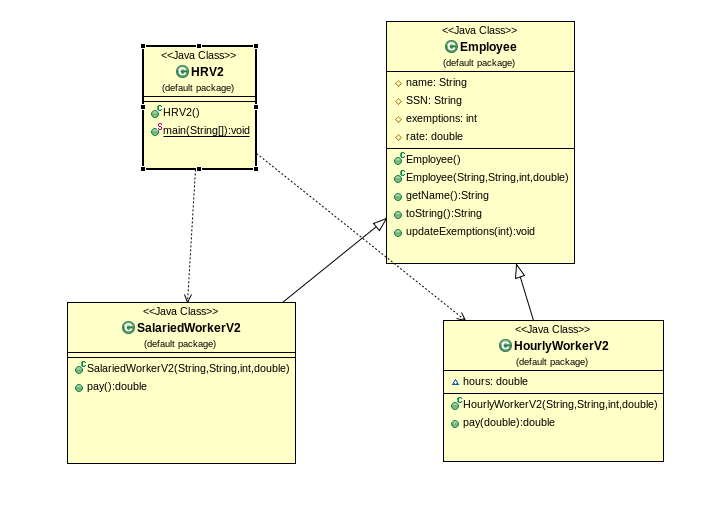
This UML describes the better solution that you will explore in this section. Notice the arrows pointing from the children back to the parent.
Download the 4 classes, Employee.java, SalariedWorkerV2.java,
HourlyWorkerV2.java and HRV2.java.
- Look at the list of instance
variables (attributes) that
you created in question 4.1. In
which class are these located?
- Are
these attributes found anywhere
in any of the other
three classes?
- Look at the SalariedWorkerV2.java
class. Does it
contain any attributes of its own?
- What
do you see that might "link" SalariedWorkerV2.java
to
the Employee.java class?
- How
about HourlyWorkerV2.java?
Does it contain any
attributes? Does it also link to the Employee.java
class?
- Review: The extends keyword in java
tells the compiler that this
class is related to a parent. The parent is identified in the
extends clause in the class header. We say that SalariedWorkerV2 and HourlyWorkerV2
are children of or subclasses of
the Employee class. Employee.java is the superclass or
parent of the other two. Both SalariedWorkerV2
and HourlyWorkerV2 are specializations of
the
general employee class and we
can also say that SalariedWorkerV2 is-a Employee and HourlyWorkerV2
is-a Employee..
- In HRV2.java,
do you see any objects of the
"Employee" type?
- In HRV2.java, we are building
the more specific versions of Employee,
either an HourlyWorkerV2 or SalariedWorkerV2. Looking
at the methods in Employee, what method does not exist in Employee.java
that does exist in each of the two specializations?
- What methods exists in Employee.java that do not exist in each of the two specializations?
- Does
this make sense? Why or why not?
- Notice that the HourlyWorker
and SalariedWorker constructors
accept the same parameters as they did before. Somehow we
need to
transmit those parameters to the Employee
class where the attributes
are actually located. super is the
keyword to identify the
parent
class just as this is used to identify a class within itself.
You
call the parent constructor by calling super
and passing in the
appropriate parameters. Do this for the SalariedWorkerV2
and HourlyWorkerV2 classes.
- Compile the superclass and
each of the subclasses. Compile the driver and test.
Notice that the toString works even
though we have not
created an Employee object. Each of
the two
subclasses use its parent's
method unless they have one of their own.
- Notice that pay() has not
been defined for either of the
subclasses. Create appropriate pay()
methods based on your
final
work with SalariedWorker.java and HourlyWorker.java. Compile
and
test.
6 Extending the inheritance:
In part 4, you added a field to each of the classes.
- Where
would you add this same field to your Employee.java
family of classes?
Where would you change the toString()?
What constructors do
you need to change?
- Do this and test your
solution.
- Does inheritance save you any
coding?
What would you say are the advantages and disadvantages of
using
an inheritance structure for a related set of classes?
7 Polymorphism:
- Copy HRV2.java to HRV3.java.
- Using the Polymorphism feature of Java (many forms) we could potentially get rid of the calls to individual objects if we could group them into an array since we are doing most of the processing in a group.
- Create an array of Employee at the beginning of the main method and instantiate it to 4 elements. Assign each of the objects (the SalariedWorkerV2 and HourlyWorkerV2) to a corresponding array element (0-3).
- Change each of the groups of processes so that instead of printing or paying individual employees we are printing or paying them all as a group using a for loop.
- Compile the HRV3.java program. What error do you get?
- Since the pay method is not in Employee, the compiler does not know about the pay methods. Add a pay method to Employee that returns 0. Compile the HRV3.java program. Do you get any errors?
- Run the HRV3.java program. Are the results correct (consistent with the first version)? Why or why not?
- How might you solve this problem? In other words, can you design a solution that will enable us to pay all employees at once?
- Be prepared to discuss in class next class period.
Turn in this assignment by the preferred method from your instructor.
Updated 04/10/2014 (nlh)